We have three types of array in PHP
- Index arrays
- associative arrays
- multidimensional arrays
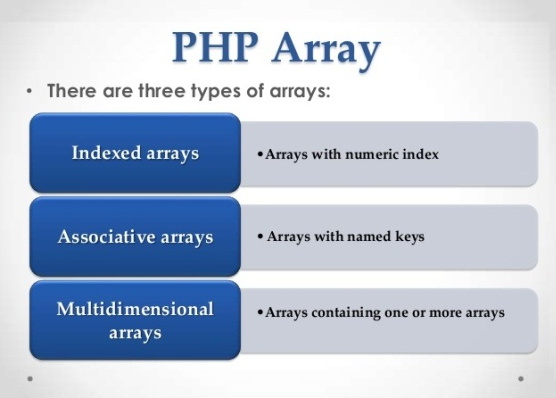
1. Index Array (Numeric Array)
These arrays can store numbers, strings and any object but their index will be represented by numbers. By default array index starts from zero.
We can use index array in two ways
First way
<?php
// first way
$subjects=array("c++","php","java","os");
echo " subjects: $c++[0],$php[1],$java[2],$os[3]";
?>
output
subjects: c++,php,java,os
Second way
<?php
// second way
$subjects[0]="c++";
$subjects[1]="php";
$subjects[2]="java";
$subjects[3]="os";
echo "subjects: $c++[0],$php[1],$java[2],$os[3]";
?>
output
subjects: c++,php,java,os
2. Associative Array
These types of arrays are similar to the indexed arrays but instead of linear storage, every value can be assigned with a user-defined key of string type.
Associative array is also used in two ways
First way to use associative array
<?php
// first way
$marks=array("c++"=>"23","php"=>"24","java"=>"25");
echo "c++: ".$marks["c++"]."<br/>";
echo "php: ".$marks["phpjava"]."<br/>";
echo "java: ".$marks["Kartik"]."<br/>";
?>
output
c++:23
php:24
java:25
Second wat to use associative array
<?php
// second way
$marks["c++"]="23";
$marks["php"]="24";
$marks["java"]="25";
echo "c++ ".$marks["c++"]."<br/>";
echo "php ".$marks["php"]."<br/>";
echo "java ".$marks["java"]."<br/>";
?>
output
c++ 23
php 24
java 25
3. Multidimensional Array
A multi-dimensional array each element in the main array can also be an array. And each element in the sub-array can be an array, and so on. Values in the multi-dimensional array are accessed using multiple index.
exmple
<?php
$marks = array(
"c++" => array (
"practicals" => 22,
"internal1" => 23,
"internal2" => 24
),
"php" => array (
"practicals" => 23,
"internal1" => 24,
"internal2" => 25
),
"java" => array (
"practicals" => 25,
"internal1" => 26,
"internal2" => 27
)
);
echo "Marks for c++ in practicals : " ;
echo $marks['c++']['practicals'] . "<br />";
echo "Marks for php in internal1 : ";
echo $marks['php']['internal1'] . "<br />";
echo "Marks for java in internal2 : " ;
echo $marks['java']['internal2'] . "<br />";
?>
output
Marks for c++ in practicals : 22
Marks for php in internal1 : 24
Marks for java in internal2 : 27