There are multiple different types of conditionals statement
- “if” statement
- “if else ” statement
- “if else if” statement
“if” statement
The if statement is the fundamental control statement that allows JavaScript to make decisions and execute statements conditionally.
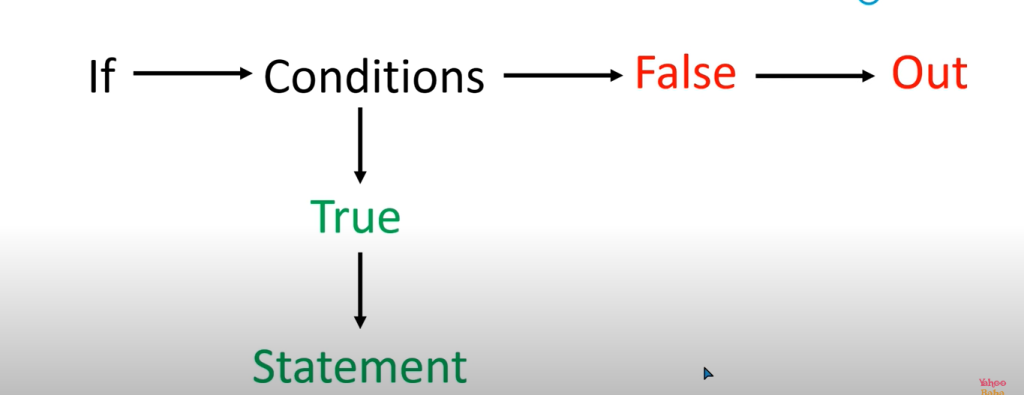
synatx
if (expression)
{
Statement
}
“if else” statement
The ‘if…else’ statement is the next form of control statement that allows JavaScript to execute statements in a more controlled way.
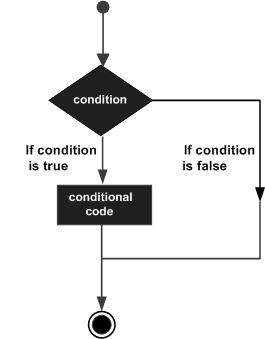
syntax
if (expression)
{
Statement
} else
{
Statement
}
“if else if “statement
The if…else if… statement is an advanced form of if…else that allows JavaScript to make a correct decision out of several conditions.
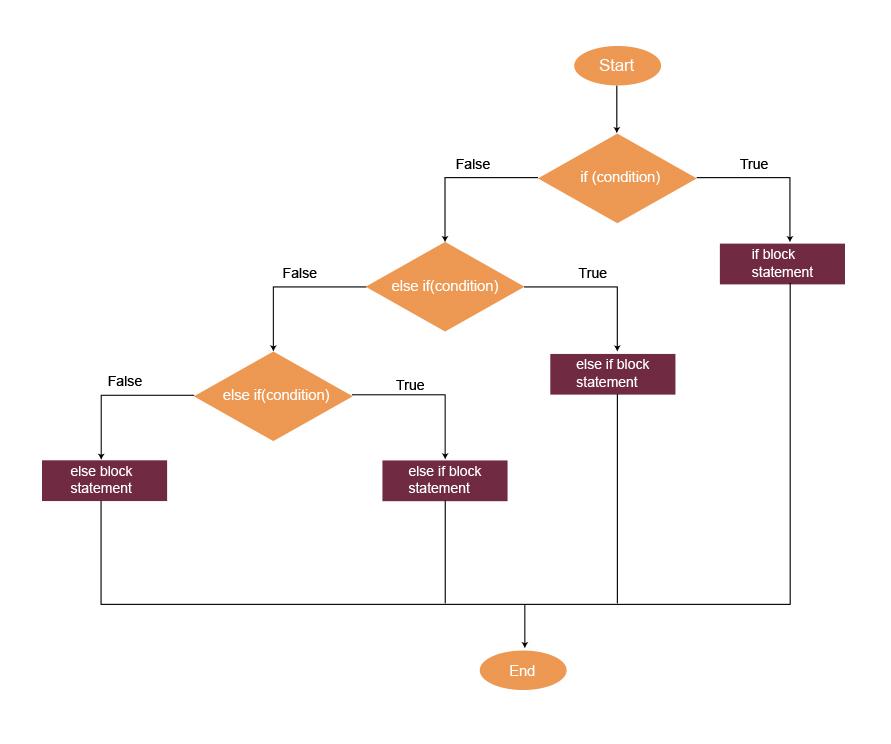
syntax
if (expression 1)
{
Statement
} else if (expression 2)
{
Statement
} else if (expression 3)
{
Statement
} else
{
Statement
}
example
<!DOCTYPE html>
<html>
<head>
<title>javascript</title>
</head>
<body>
<script>
var a=200;
var b=300;
if(a<b)
{
document.write("a is less than b");
}
else if(a>b)
{
document.write("a is greater than b");
}
else if(a==b)
{
document.write("a is equal to b");
}
else
{
document.write("wrong value");
}
</script>
</body>
</html>
output
a is less than b